Alright, let’s talk about this “genesis leaderboard” thing I’ve been messing with. It’s been a journey, not gonna lie.
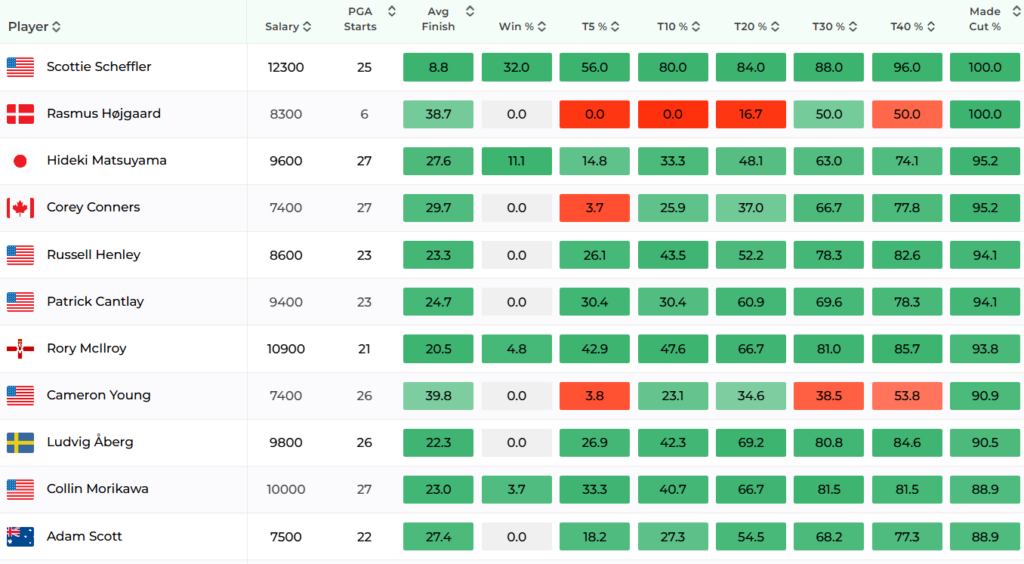
First off, I started with a basic idea: I wanted to track some kind of performance metric within a system I was building. Think of it like a scoreboard, but for… well, let’s just say “achievements.” I didn’t want anything fancy, just a simple list, sorted by score, with names attached.
So, step one: data structure. I went with a straightforward approach: a list of dictionaries. Each dictionary had a ‘name’ key and a ‘score’ key. Easy peasy.
Next, I needed a way to add scores. I created a function called `update_leaderboard(name, score)`. This function would:
- Check if the ‘name’ already existed in the leaderboard.
- If it did, it would update the score.
- If it didn’t, it would add a new entry to the leaderboard.
Pretty basic, right?
The real challenge came with sorting. I wanted the leaderboard to be sorted by score in descending order. So, I used Python’s `sorted()` function with a `lambda` function as the key. The `lambda` function simply returned the ‘score’ of each entry. Boom, sorted!
Now, here’s where things got a little messy. I realized I needed to persist the leaderboard data. Just keeping it in memory wasn’t gonna cut it. So, I decided to use a simple text file. Each line in the file would represent a leaderboard entry, with the name and score separated by a comma.
I added functions to load the leaderboard from the file and save it back to the file. The loading function would read each line, split it into name and score, and add it to the leaderboard list. The saving function would iterate through the leaderboard list and write each entry to the file in the correct format.
Then I thought, “What if multiple people are trying to update the leaderboard at the same time?” That’s a recipe for disaster! So, I added a simple file locking mechanism using the `fcntl` module (on Linux, anyway. Had to use some `msvcrt` on Windows. Ugh). This made sure only one process could access the leaderboard file at a time.
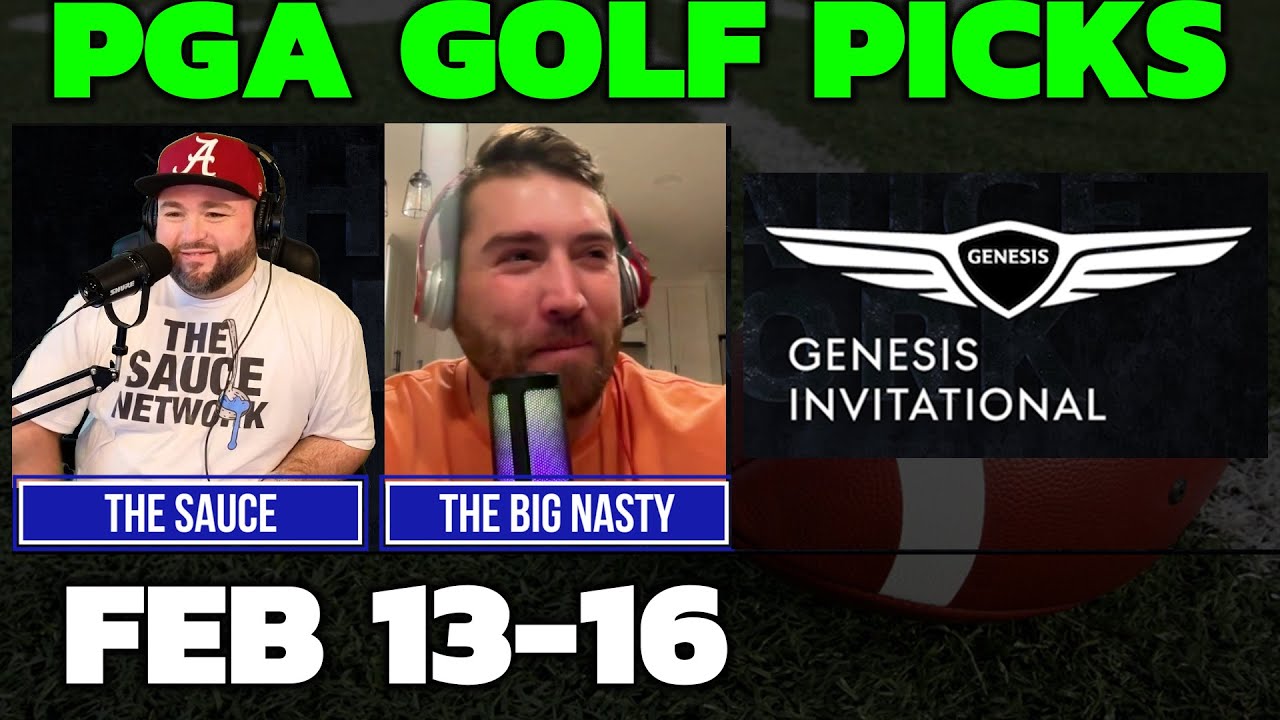
After that, I started playing around with how I wanted to display the leaderboard. I used some basic string formatting to create a nice, clean output. I also added a limit to the number of entries displayed, so the leaderboard wouldn’t get too long.
The final piece was adding some error handling. I wrapped the file operations in `try…except` blocks to catch any potential errors, like the file not existing or being corrupted. I also added some basic input validation to make sure the ‘score’ was actually a number.
And that’s pretty much it! A simple, functional leaderboard. It’s not the most elegant solution, but it gets the job done. The whole thing probably took me a couple of evenings to hammer out, mostly because I kept getting tripped up by silly syntax errors.
Here’s a rough outline of the code structure:
- `update_leaderboard(name, score)`: Updates the leaderboard with a new score.
- `get_leaderboard()`: Returns the sorted leaderboard list.
- `load_leaderboard_from_file()`: Loads the leaderboard from a text file.
- `save_leaderboard_to_file()`: Saves the leaderboard to a text file.
- `display_leaderboard(limit=10)`: Displays the leaderboard in a formatted way.
And a few lessons learned:
- File locking is crucial when dealing with shared data.
- Simple solutions are often the best solutions.
- Error handling is your friend.
Overall, this “genesis leaderboard” project was a fun little exercise. It helped me brush up on my basic Python skills and learn a few new tricks. And hey, now I have a working leaderboard system that I can use in future projects. Not bad for a couple of evenings’ work!